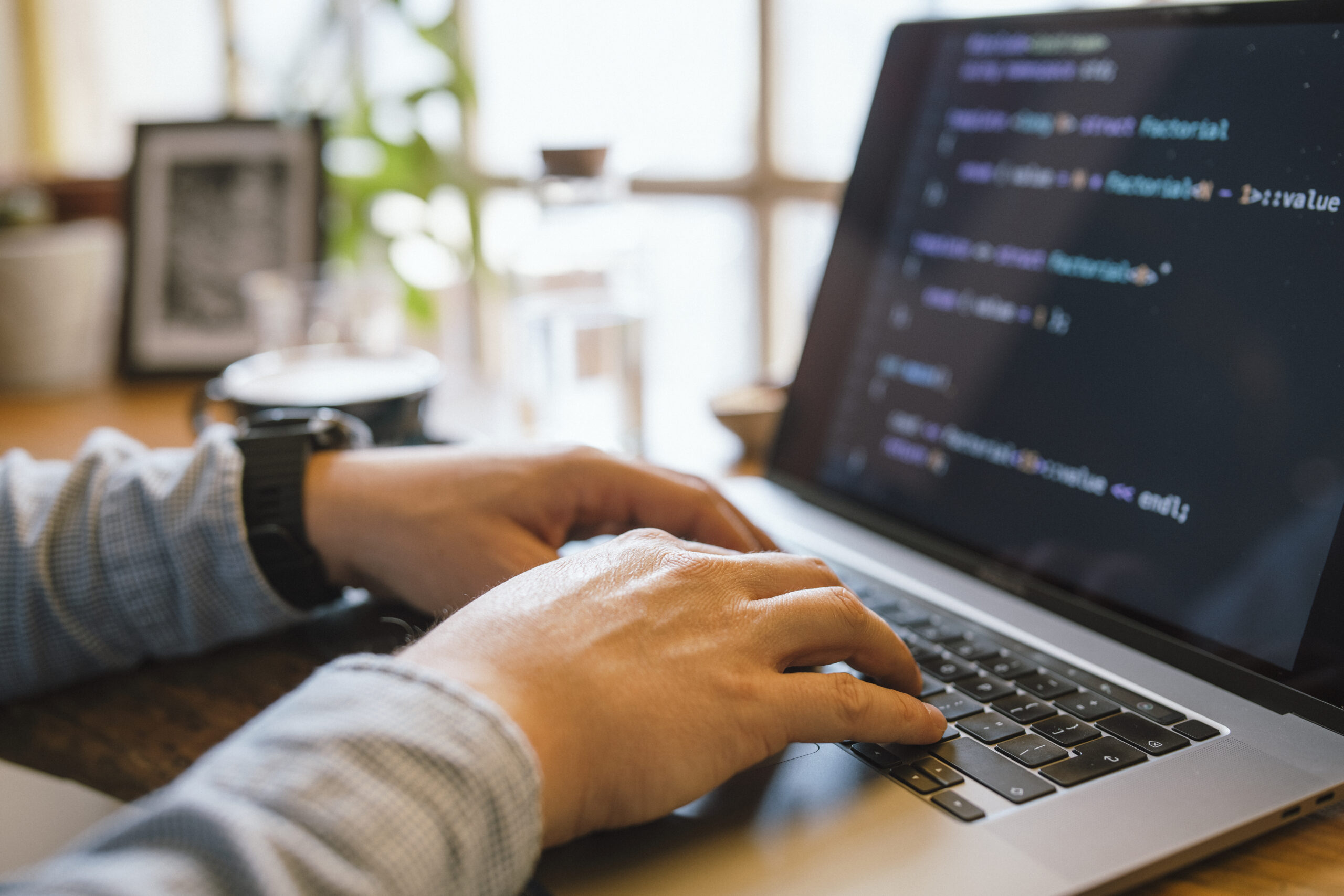
Debugging is Among the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Consider methodically to resolve troubles successfully. Irrespective of whether you are a rookie or a seasoned developer, sharpening your debugging skills can save hours of frustration and dramatically improve your productiveness. Listed below are numerous methods to assist builders amount up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single A part of advancement, understanding how to connect with it properly throughout execution is Similarly critical. Modern-day advancement environments come Geared up with effective debugging abilities — but a lot of builders only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step through code line by line, and in many cases modify code within the fly. When made use of accurately, they let you notice just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-close developers. They help you inspect the DOM, keep track of community requests, perspective actual-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch frustrating UI concerns into workable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage around operating processes and memory administration. Discovering these resources could have a steeper Mastering curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command systems like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic alterations.
Ultimately, mastering your tools indicates going over and above default options and shortcuts — it’s about establishing an personal expertise in your development environment to ensure that when concerns come up, you’re not misplaced at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the particular challenge rather then fumbling by the method.
Reproduce the challenge
The most essential — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or making guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question inquiries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances underneath which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local ecosystem. This might imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions associated. These tests not merely assistance expose the trouble but additionally protect against regressions in the future.
At times, the issue could be natural environment-specific — it would come about only on sure operating units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical approach. But when you can constantly recreate the bug, you are by now midway to correcting it. That has a reproducible state of affairs, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse extra Evidently with your workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Read and Comprehend the Mistake Messages
Mistake messages in many cases are the most respected clues a developer has when some thing goes wrong. Rather than looking at them as disheartening interruptions, builders must discover to deal with error messages as immediate communications with the program. They usually tell you what precisely took place, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into components. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it level to a selected file and line amount? What module or functionality activated it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some errors are obscure or generic, As well as in Those people instances, it’s important to look at the context by which the error happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most highly effective applications inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an software behaves, encouraging you understand what’s taking place under the hood without needing to pause execution or step through the code line by line.
A great logging technique starts with knowing what to log and at what amount. Typical logging ranges consist of DEBUG, INFO, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details through growth, Data for basic occasions (like successful get started-ups), Alert for likely concerns that don’t split the application, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with too much or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, for instance timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively recognize and take care of bugs, developers should strategy the method similar to a detective resolving a secret. This mindset assists break down elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as you can with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, sort hypotheses. Check with on your own: What may very well be triggering this conduct? Have any adjustments lately been produced to the codebase? Has this issue occurred before less than related conditions? The objective is to slender down options and recognize possible culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out near attention to compact aspects. Bugs usually hide during the minimum expected areas—similar to a missing semicolon, an off-by-one particular mistake, or maybe a race issue. Be thorough and individual, resisting the urge to patch The difficulty with out thoroughly comprehending it. Non permanent fixes may possibly disguise the real trouble, only for it to resurface later on.
Lastly, hold notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Produce Exams
Composing assessments is among the most effective approaches to increase your debugging competencies and overall advancement effectiveness. Assessments not simply aid catch bugs early but in addition function a security Internet that offers you assurance when making modifications in your codebase. A effectively-examined software is much easier to debug mainly because it helps you to pinpoint exactly exactly where and when an issue occurs.
Start with click here unit checks, which focus on individual features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a particular piece of logic is working as expected. Any time a exam fails, you promptly know where to look, significantly lessening some time expended debugging. Unit tests are especially useful for catching regression bugs—challenges that reappear immediately after Earlier staying mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software get the job done alongside one another easily. They’re especially practical for catching bugs that come about in sophisticated systems with various elements or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to think critically about your code. To check a attribute properly, you require to comprehend its inputs, envisioned outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. After the take a look at fails regularly, you may focus on repairing the bug and enjoy your test move when The difficulty is fixed. This strategy makes sure that the same bug doesn’t return Later on.
To put it briefly, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult issue, it’s straightforward to become immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps commence overlooking apparent mistakes or misreading code which you wrote just hours earlier. In this state, your Mind will become a lot less successful at challenge-fixing. A short walk, a coffee crack, or simply switching to a unique undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the basis of an issue when they've taken the perfect time to disconnect, allowing their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent helps you to return with renewed Strength as well as a clearer mindset. You would possibly out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline is to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver all around, extend, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, but it surely really leads to more rapidly and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than just a temporary setback—It truly is a possibility to grow for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you one thing worthwhile for those who take the time to reflect and examine what went Mistaken.
Start out by inquiring yourself a couple of crucial thoughts as soon as the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like device screening, code testimonials, or logging? The solutions typically expose blind spots with your workflow or comprehension and make it easier to Make stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring challenges or prevalent problems—you could proactively prevent.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Some others stay away from the identical problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential portions of your improvement journey. In fact, several of the best builders aren't those who compose perfect code, but individuals who continuously study from their errors.
In the long run, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.